PALINDROME STRING IN PYTHON: To find weather string is Palindrome or not firstly we must know what is Palindrome String is ? Palindrome is string which reverse is equal to original string. for example string “MADAM” , reverse of string MADAM is MADAM hence this string is called palindrome string. Take another example a student named NITIN is palindrome string because reverse of NITIN is NITIN. So now task is to find the string whether its palindrome of not.
FIND PALINDROME STRING IN PYTHON CODE NUMBER 1
str1=input("Enter String=")
str2=str1[::-1] # Reverse String using String slicing
print("Orignal String=",str1)
print("Reverse is =",str2)
if str1==str2:
print("String is plaindrome")
else:
print("String is not Plaindrome")
- Take a String or Input String
- Store reverse of that string in another string.
- Now compare both string for equality
- if equal then Print String is Palindrome
- if not then Print String is not Palindrome.
in above code we use string slicing concept of Python. In String Slicing the Slice[n : m] operator extracts sub parts from the strings. for example if
A="Save Earth"
print(A[1:3])
av
# The print statement prints the substring starting from subscript 1 and ending at
subscript 3 .
More on string Slicing Consider the given figure
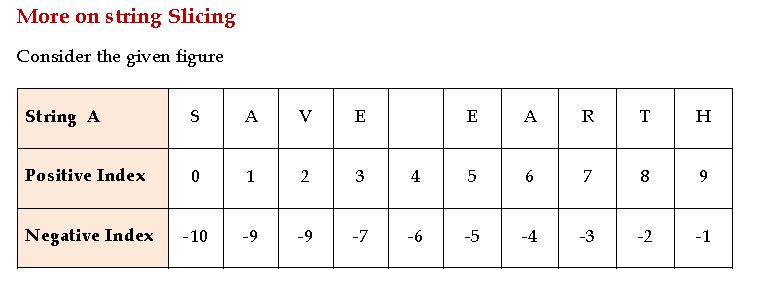
print A[3:] will print ‘e Earth’
Omitting the second index, directs the python interpreter to extract the substring till the end of the string.
print A[:3] will print Sav.
Omitting the first index, directs the python interpreter to extract the substring before the second index starting from the beginning.
print A[:] will Print Save Earth
Omitting both the indices, directs the python interpreter to extract the entire string starting from 0 till the last index.
print A[-2:] will print th
For negative indices the python interpreter counts from the right side (also shown above). So the last two letters are printed.
print A[:-2] will print Save Ear
Omitting the first index, directs the python interpreter to start extracting the substring form the beginning. Since the negative index indicates slicing from the end of the string. So the entire string except the last two letters is printed.
you can watch more about String Slicing in Python by clicking video given below.
Alternate Code to find palindrome string in Python.
str1=input("Enter String=")
str2=""
for i in range(len(str1)-1,-1,-1):
str2=str2+str1[i]
print("orignal string ",str1)
print("reverse string ",str2)
if str1==str2:
print("palindrome string")
else:
print("not palindrome string")
str1=input("Enter String=")
str2=""
k=-1
for i in range(len(str1)):
str2=str2+str1[k]
k=k-1
print("orignal String ", str1)
print("Reverse is ",str2)
if str1==str2:
print("String is palindrome")
else:
print("String is not palindrome")